Table of Contents
Are you struggling to integrate .NET Core with Angular in your web development projects? 🤔 You’re not alone. Many developers find themselves lost in the maze of these two powerful frameworks, unsure how to make them work seamlessly together.
Imagine building a robust, scalable web application that harnesses the server-side prowess of .NET Core and the dynamic, responsive UI capabilities of Angular. Sounds exciting, right? 🚀 But the path to this perfect union can be fraught with challenges – from setting up the development environment to deploying the final product.
Fear not! In this comprehensive guide, we’ll walk you through the entire process of integrating .NET Core with Angular. We’ll cover everything from understanding the basics to advanced topics like authentication, performance optimization, and DevOps practices. Whether you’re a seasoned developer or just starting out, this guide will equip you with the knowledge and tools to create cutting-edge web applications. Let’s dive in and explore the world of .NET Core and Angular integration!
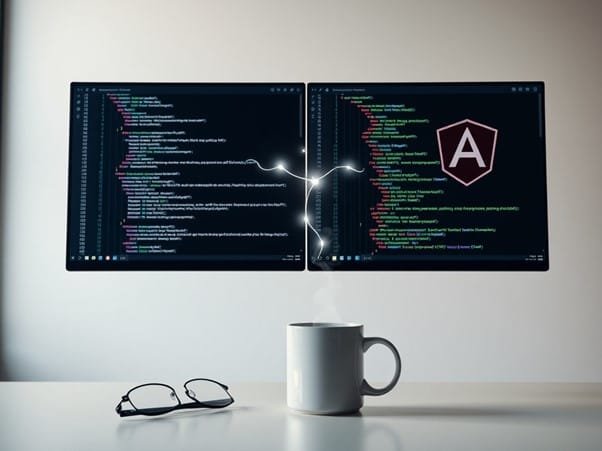
Understanding .NET Core and Angular
Key features of .NET Core
.NET Core is a powerful, open-source framework developed by Microsoft. It offers several key features that make it an excellent choice for modern web development:
- Cross-platform compatibility
- High performance
- Modular architecture
- Side-by-side versioning
- CLI tools for easy development
Angular framework basics
Angular is a popular frontend framework maintained by Google. It provides developers with a robust set of tools for building dynamic, single-page applications:
- Component-based architecture
- Two-way data binding
- Dependency injection
- Powerful CLI for project management
- Comprehensive testing utilities
Benefits of integrating .NET Core with Angular
Combining .NET Core and Angular creates a powerful full-stack solution with numerous advantages:
Benefit | Description |
Separation of concerns | Clear division between backend and frontend |
Scalability | Both frameworks are designed to handle large-scale applications |
Performance | .NET Core’s speed complements Angular’s efficient rendering |
Developer productivity | Shared concepts and tooling across both technologies |
Prerequisites for integration
Before integrating .NET Core with Angular, ensure you have the following:
- .NET Core SDK (latest version)
- Node.js and npm
- Angular CLI
- Visual Studio Code or Visual Studio (IDE)
- Basic knowledge of C# and TypeScript
With these components in place, you’re ready to begin the integration process. In the next section, we’ll dive into setting up your development environment for a seamless .NET Core and Angular project.
Setting Up the Development Environment
Now that we understand the basics of .NET Core and Angular, let’s dive into setting up our development environment. This crucial step will ensure we have all the necessary tools and configurations in place to build our integrated application.
1. Installing .NET Core SDK
To begin our development journey, we need to install the .NET Core SDK. This software development kit provides all the tools necessary to build and run .NET Core applications.
- Visit the official Microsoft .NET download page
- Choose the appropriate version for your operating system
- Download and run the installer
- Follow the installation wizard instructions
- Verify the installation by opening a terminal and running: dotnet –version
2. Setting up Node.js and npm
Node.js and npm (Node Package Manager) are essential for Angular development. Here’s how to set them up:
- Go to the official Node.js website
- Download the LTS (Long Term Support) version for your OS
- Run the installer and follow the prompts
- Verify the installation by opening a terminal and running:
- node –version
- npm –version
3. Installing Angular CLI
Angular CLI (Command Line Interface) is a powerful tool for creating and managing Angular projects. Let’s install it:
- Open a terminal or command prompt
- Run the following command: npm install -g @angular/cli
- Verify the installation by running: ng version
4. Configuring IDE for .NET Core and Angular development
Choosing the right Integrated Development Environment (IDE) can significantly boost your productivity. Here’s a comparison of popular IDEs:
IDE | .NET Core Support | Angular Support | Free Version |
Visual Studio | Excellent | Good | Community Edition |
Visual Studio Code | Good | Excellent | Yes |
JetBrains Rider | Excellent | Excellent | 30-day trial |
For this guide, we recommend Visual Studio Code due to its lightweight nature and excellent support for both .NET Core and Angular. To configure it:
- Download and install Visual Studio Code
- Install the following extensions:
- C# for Visual Studio Code
- Angular Language Service
- Angular Snippets
- Configure settings for optimal development experience
With these steps completed, your development environment is now ready for integrating .NET Core with Angular. Next, we’ll move on to creating our .NET Core Web API.
Creating a .NET Core Web API
Now that we’ve set up our development environment, let’s dive into creating a robust .NET Core Web API. This crucial step will serve as the backend for our Angular application.
1. Initializing a new .NET Core project
To begin, open your terminal and run the following command to create a new .NET Core Web API project:
dotnet new webapi -n MyAngularNetCoreApp
This command initializes a new project with the basic structure and files needed for a Web API.
2. Implementing API controllers
API controllers are the heart of your Web API. They handle incoming HTTP requests and return appropriate responses. Here’s a simple example of a controller:
[ApiController]
[Route("api/[controller]")]
public class WeatherForecastController : ControllerBase
{
[HttpGet]
public IEnumerable Get()
{
// Implementation details
}
}
3. Setting up data models and services
Data models represent the structure of your data, while services contain the business logic. Here’s a basic example:
public class WeatherForecast
{
public DateTime Date { get; set; }
public int TemperatureC { get; set; }
public string Summary { get; set; }
}
public interface IWeatherService
{
IEnumerable GetForecasts();
}
public class WeatherService : IWeatherService
{
public IEnumerable GetForecasts()
{
// Implementation details
}
}
4. Configuring CORS for Angular integration
To allow your Angular app to communicate with your API, you need to configure Cross-Origin Resource Sharing (CORS). Add the following to your Startup.cs file:
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy("AllowAngularOrigins",
builder =>
{
builder.WithOrigins("http://localhost:4200")
.AllowAnyHeader()
.AllowAnyMethod();
});
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseCors("AllowAngularOrigins");
}
5. Testing the API endpoints
Finally, it’s crucial to test your API endpoints. You can use tools like Postman or Swagger UI, which comes built-in with .NET Core Web API projects. Here’s a comparison of these tools:
Feature | Postman | Swagger UI |
GUI | Standalone application | Built into .NET Core |
API Documentation | Manual | Automatic |
Testing Complexity | Advanced | Basic |
Learning Curve | Moderate | Low |
With your .NET Core Web API now set up and tested, we’re ready to move on to building the Angular frontend that will consume this API.
Building the Angular Frontend
Now that we have our .NET Core Web API set up, let’s focus on creating the Angular frontend to consume our API and provide a rich user interface.
Generating a new Angular project
To start building our Angular frontend, we’ll use the Angular CLI to generate a new project. Open your terminal and run:
ng new my-angular-app
cd my-angular-app
This command creates a new Angular project with a basic structure and installs all necessary dependencies.
Creating components and services
Angular’s component-based architecture allows for modular and reusable code. Let’s create some essential components and services:
ng generate component home
ng generate component user-list
ng generate service api
These commands will create a home component, a user-list component, and an API service to handle HTTP requests to our .NET Core backend.
Implementing routing
Routing in Angular enables navigation between different views. Add the following routes to your app-routing.module.ts:
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'users', component: UserListComponent },
];
Designing responsive UI with Angular Material
Angular Material provides pre-built UI components that follow Material Design principles. Install it using:
ng add @angular/material
Now, let’s compare some popular Angular UI libraries:
Library | Pros | Cons |
Angular Material | Official Google library, extensive documentation | Opinionated design, larger bundle size |
PrimeNG | Rich set of components, theming support | Steeper learning curve |
NGX-Bootstrap | Bootstrap-based, familiar design | Limited custom styling options |
Choose the library that best fits your project requirements and design preferences.
With these steps completed, we have a solid foundation for our Angular frontend. Next, we’ll see how to connect this frontend to our .NET Core API for seamless data flow and interaction.
Connecting Angular to .NET Core API
Now that we have our .NET Core Web API and Angular frontend set up, it’s time to establish the connection between them. This crucial step enables our Angular application to communicate with the backend and retrieve or send data as needed.
Configuring HttpClient in Angular
To begin, we need to configure HttpClient in our Angular application. This powerful module allows us to make HTTP requests to our .NET Core API.
1. Import HttpClientModule in app.module.ts:
import { HttpClientModule } from '@angular/common/http';
@NgModule({
imports: [
// other imports
HttpClientModule
],
// ...
})
export class AppModule { }
2. Inject HttpClient in your service:
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class ApiService {
constructor(private http: HttpClient) { }
}
Creating service methods for API calls
With HttpClient configured, we can now create methods in our service to interact with the API:
@Injectable({
providedIn: 'root'
})
export class ApiService {
private apiUrl = 'https://your-api-url.com/api';
constructor(private http: HttpClient) { }
getData(): Observable {
return this.http.get(`${this.apiUrl}/data`);
}
postData(data: any): Observable {
return this.http.post(`${this.apiUrl}/data`, data);
}
}
Implementing error handling and data transformation
Error handling and data transformation are crucial for robust API integration:
import { catchError, map } from 'rxjs/operators';
import { throwError } from 'rxjs';
getData(): Observable {
return this.http.get(`${this.apiUrl}/data`).pipe(
map(response => this.transformData(response)),
catchError(this.handleError)
);
}
private transformData(data: any): any {
// Transform data as needed
return data;
}
private handleError(error: HttpErrorResponse) {
let errorMessage = 'An error occurred';
if (error.error instanceof ErrorEvent) {
// Client-side error
errorMessage = `Error: ${error.error.message}`;
} else {
// Server-side error
errorMessage = `Error Code: ${error.status}\nMessage: ${error.message}`;
}
console.error(errorMessage);
return throwError(errorMessage);
}
Using observables for real-time data updates
Observables provide a powerful way to handle asynchronous data and real-time updates:
Feature | Description |
Subscription | Subscribe to observables to receive data updates |
Unsubscription | Always unsubscribe to prevent memory leaks |
Operators | Use RxJS operators for data manipulation |
Example usage in a component:
export class DataComponent implements OnInit, OnDestroy {
private dataSubscription: Subscription;
constructor(private apiService: ApiService) { }
ngOnInit() {
this.dataSubscription = this.apiService.getData().subscribe(
data => console.log(data),
error => console.error(error)
);
}
ngOnDestroy() {
if (this.dataSubscription) {
this.dataSubscription.unsubscribe();
}
}
}
With these implementations, your Angular application is now effectively connected to your .NET Core API, enabling seamless data exchange and real-time updates. Next, we’ll explore how to implement authentication and authorization to secure this connection.
Authentication and Authorization
Authentication and authorization are crucial aspects of any web application, ensuring that users can securely access their data and perform authorized actions. In this section, we’ll explore how to implement these security measures in our .NET Core and Angular integration.
Implementing JWT Authentication in .NET Core
JSON Web Tokens (JWT) provide a secure and efficient way to authenticate users in .NET Core applications. Here’s how to implement JWT authentication:
- Install the necessary NuGet packages:
- Microsoft.AspNetCore.Authentication.JwtBearer
- System.IdentityModel.Tokens.Jwt
- Configure JWT settings in appsettings.json:
{
"Jwt": {
"Key": "your-secret-key",
"Issuer": "your-issuer",
"Audience": "your-audience"
}
}
    3. Set up JWT authentication in Startup.cs:
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options => {
// Configure JWT options
});
Creating Login and Registration Components in Angular
To enable user authentication on the frontend, we need to create login and registration components:
- Generate components:
- ng generate component login
- ng generate component register
- Implement forms for user input and connect to authentication service.
- Create an authentication service to handle API calls:
@Injectable({
providedIn: 'root'
})
export class AuthService {
login(credentials: any): Observable {
// Implement login logic
}
register(user: any): Observable {
// Implement registration logic
}
}
Securing API Endpoints with Authorization
To protect sensitive endpoints in your .NET Core API:
- Use the [Authorize] attribute on controllers or actions:
[Authorize]
[ApiController]
[Route("api/[controller]")]
public class SecureController : ControllerBase
{
// Secure endpoints
}
    2. Implement role-based authorization for fine-grained control:
[Authorize(Roles = "Admin")]
public IActionResult AdminOnlyEndpoint()
{
// Admin-only logic
}
Managing User Sessions and Token Storage
Proper token management is essential for maintaining secure user sessions:
Storage Method | Pros | Cons |
Local Storage | Persists across sessions | Vulnerable to XSS attacks |
HTTP-only Cookies | More secure against XSS | Requires additional server-side logic |
In-memory Storage | Secure, cleared on page refresh | Doesn’t persist across sessions |
Implement a token interceptor in Angular to automatically add the token to API requests:
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
intercept(request: HttpRequest, next: HttpHandler): Observable> {
// Add token to request headers
return next.handle(request);
}
}
By implementing these authentication and authorization measures, you’ll significantly enhance the security of your .NET Core and Angular application. Next, we’ll explore techniques for optimizing the performance of your integrated application to ensure it runs smoothly and efficiently.
Optimizing Performance
Now that we’ve covered the basics of integration, let’s focus on optimizing the performance of our .NET Core and Angular application. Performance optimization is crucial for delivering a smooth user experience and improving overall efficiency.
1. Implementing lazy loading in Angular
Lazy loading is a powerful technique in Angular that can significantly improve your application’s initial load time. By breaking your app into feature modules and loading them on-demand, you can reduce the initial bundle size and speed up the initial rendering.
Here’s a simple example of how to implement lazy loading:
const routes: Routes = [
{ path: 'home', component: HomeComponent },
{ path: 'products', loadChildren: () => import('./products/products.module').then(m => m.ProductsModule) }
];
2. Caching strategies for .NET Core API
Implementing effective caching strategies in your .NET Core API can dramatically reduce server load and improve response times. Here are some popular caching options:
Caching Strategy | Description | Best Use Case |
In-Memory Caching | Stores data in the application’s memory | Frequently accessed, small-sized data |
Distributed Caching | Stores data across multiple servers | Large-scale applications with multiple instances |
Response Caching | Caches entire HTTP responses | Static or semi-static content |
3. Minimizing payload size with compression
Compressing API responses can significantly reduce data transfer times. .NET Core provides built-in support for response compression. Enable it in your Startup.cs:
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression();
}
public void Configure(IApplicationBuilder app)
{
app.UseResponseCompression();
}
4. Utilizing server-side rendering for improved SEO
Server-side rendering (SSR) can enhance your application’s SEO and initial load performance. Angular Universal allows you to run your Angular app on the server, generating static content for better indexing by search engines.
To implement SSR:
- Install necessary packages
- Create a server-side app module
- Modify your main.ts and main.server.ts files
- Update your angular.json configuration
By implementing these optimization techniques, you’ll significantly improve your application’s performance, providing a better user experience and potentially boosting your search engine rankings. In the next section, we’ll explore testing and debugging strategies to ensure your optimized application functions correctly.
Testing and Debugging
Now that we’ve optimized our application’s performance, it’s crucial to ensure its reliability through comprehensive testing and debugging. This section will cover various testing strategies and debugging techniques for our full-stack .NET Core and Angular application.
A. Unit testing .NET Core API
Unit testing is essential for maintaining the integrity of our .NET Core API. Here’s a brief overview of how to approach unit testing:
- Use xUnit or NUnit as your testing framework
- Create test classes for each controller or service
- Write tests for individual methods and edge cases
- Utilize mocking frameworks like Moq for isolating dependencies
Example of a simple unit test using xUnit:
public class UserControllerTests
{
[Fact]
public async Task GetUser_ReturnsCorrectUser()
{
// Arrange
var mockRepo = new Mock();
mockRepo.Setup(repo => repo.GetUserByIdAsync(1))
.ReturnsAsync(new User { Id = 1, Name = "John Doe" });
var controller = new UserController(mockRepo.Object);
// Act
var result = await controller.GetUser(1);
// Assert
var okResult = Assert.IsType(result);
var user = Assert.IsType(okResult.Value);
Assert.Equal(1, user.Id);
Assert.Equal("John Doe", user.Name);
}
}
B. Writing Angular component tests
Angular provides a robust testing framework out of the box. Here are some key points for writing effective component tests:
- Use TestBed for creating testing modules
- Isolate components by mocking services and dependencies
- Test component behavior, not implementation details
- Utilize jasmine for writing test specs
Example of an Angular component test:
describe('UserComponent', () => {
let component: UserComponent;
let fixture: ComponentFixture;
let userService: jasmine.SpyObj;
beforeEach(async () => {
const spy = jasmine.createSpyObj('UserService', ['getUser']);
await TestBed.configureTestingModule({
declarations: [ UserComponent ],
providers: [
{ provide: UserService, useValue: spy }
]
}).compileComponents();
userService = TestBed.inject(UserService) as jasmine.SpyObj;
});
beforeEach(() => {
fixture = TestBed.createComponent(UserComponent);
component = fixture.componentInstance;
});
it('should display user name', () => {
userService.getUser.and.returnValue(of({ id: 1, name: 'John Doe' }));
fixture.detectChanges();
const nameElement = fixture.nativeElement.querySelector('.user-name');
expect(nameElement.textContent).toContain('John Doe');
});
});
C. End-to-end testing with Protractor
End-to-end (E2E) testing ensures that our application functions correctly from the user’s perspective. Protractor is a popular E2E testing tool for Angular applications. Here’s a quick guide:
- Install Protractor and set up configuration
- Write test scenarios that mimic user interactions
- Use element selectors to interact with the DOM
- Run tests against different browsers and environments
Pros of E2E Testing | Cons of E2E Testing |
Simulates real user scenarios | Slower than unit tests |
Catches integration issues | More complex to set up |
Validates entire application flow | Can be flaky due to timing issues |
D. Debugging techniques for full-stack applications
Debugging a full-stack application requires a holistic approach. Here are some effective techniques:
- Use browser developer tools for frontend debugging
- Leverage Visual Studio or VS Code debugger for .NET Core
- Implement logging in both frontend and backend
- Utilize network monitoring tools to inspect API calls
Next, we’ll explore the final step in our journey: deploying our integrated .NET Core and Angular application and implementing DevOps practices for continuous improvement.
Deployment and DevOps
As we move towards the final stages of our .NET Core and Angular integration journey, let’s explore the crucial aspects of deployment and DevOps practices that will ensure smooth operation and maintenance of your application.
1. Containerizing the application with Docker
Containerization has revolutionized application deployment, and Docker is at the forefront of this technology. Here’s how to containerize your .NET Core and Angular application:
- Create separate Dockerfiles for .NET Core and Angular
- Build Docker images for each component
- Use Docker Compose to define and run multi-container applications
Component | Dockerfile Location | Base Image |
.NET Core | ./api/Dockerfile | mcr.microsoft.com/dotnet/aspnet:5.0 |
Angular | ./client/Dockerfile | node:14-alpine |
2. Continuous Integration/Continuous Deployment (CI/CD) setup
Implementing CI/CD pipelines streamlines your development process:
- Use Azure DevOps, GitLab CI, or GitHub Actions for CI/CD
- Automate build, test, and deployment processes
- Implement branch policies and code reviews
3. Deploying to cloud platforms (Azure, AWS)
Cloud platforms offer scalable and reliable hosting solutions:
- Azure App Service: Easy deployment for both .NET Core and Angular
- AWS Elastic Beanstalk: Simplified deployment and management
- Kubernetes: For more complex, scalable deployments
4. Monitoring and logging best practices
Ensure your application’s health and performance with:
- Application Insights for .NET Core
- Logging frameworks like Serilog or NLog
- Centralized logging with ELK stack (Elasticsearch, Logstash, Kibana)
- Custom Angular error handling and logging
By implementing these DevOps practices, you’ll enhance the reliability, scalability, and maintainability of your integrated .NET Core and Angular application. This approach sets a solid foundation for future growth and improvements.
Conclusion
Integrating .NET Core with Angular offers a robust and scalable solution for building modern web applications. .NET Core handles the backend logic and APIs, while Angular offers a dynamic frontend experience. With proper configuration and best practices, you can create maintainable, cross-platform, and highly efficient web apps.
FAQs
Can I use Angular with .NET Core for microservices architecture?
Yes, .NET Core is well-suited for building microservices, and Angular can be used to consume multiple APIs from different microservices to form a cohesive frontend.
How do I handle authentication in a .NET Core and Angular app?
You can use JWT (JSON Web Tokens) to manage authentication. Angular can store the token locally and send it with each request, while .NET Core can validate the token on the server.
Is it necessary to use Angular Material with Angular?
No, Angular Material is not mandatory, but it offers a variety of pre-built components that make designing responsive UIs easier.
Can I deploy both Angular and .NET Core on the same server?
Yes, by using .NET Core’s SpaServices, you can deploy both the Angular frontend and the .NET Core API on the same server.
What is the advantage of using Angular for the frontend instead of Razor Pages in .NET Core?
Angular provides a rich client-side framework with two-way data binding, reusable components, and efficient state management, making it more powerful for larger, complex applications compared to server-rendered Razor Pages.